Using SQLite with Chrome 119
Starting with Chrome 119, Google has deprecated SQlite, also referred to as WebSQL. They have been warning of this for a while. (If you're interested in why they are doing this, check out this post).
Here is how to workaround this:
Workaround 1. Use SQLite3 WASM to save to localStorage
This requires AppStudio 9.0.4 or later.
The solution is to include the SQLite3 libraries with your project. A library has been developed which provides the SQLite3 functionality for JavaScript.
AppStudio makes this easy. To enable it, select SQLite (WASM) in the Libraries section of your Project Properties:
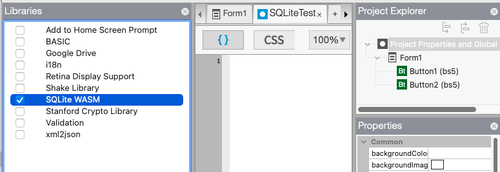
If you're using AppStudio's Sql and SqlOpenDatabase, only your SqlOpenDatabase statement will need to be changed: your database name should be localStorage to save your data between runs. It is based on kvvfs. SqlImport and sqlExport are not supported yet.
DB = SqlOpenDatabase("localStorage")
The samples SQLSample1, SQLSample2 and SQLSample3 have been updated with to use SQLite WASM.
The data saved in localStorage is persistent.
New Features
1. DB.clearStorage()
wipes out the database you created in localStorage.
2. DB.storageSize()
reports how localStorage you are using.
3. Compressing localStorage - The database in localStorage is updated whenever you update the database. However, garbage is not collected. You can clean it up (and save some space) by doing the following:
DB.clearStorage() DB.exec("VACUUM INTO 'file:local?vfs=kvvfs'")
4. The Sql function is now synchronous - meaning it executes completely before the next statement is executed. In most cases, this will make no difference to your code. We did find an error in one of our samples:
// No longer works as expected sqlList[0]=["SELECT orderno, customer FROM tabOrders;"] Sql(DB,sqlList) sqlList = [] // Proper way to do this sqlList = [] sqlList[0]=["SELECT orderno, customer FROM tabOrders;",showOrderSuccess, sqlErr] Sql(DB,sqlList)
Restrictions
- The limit on localStorage is 10 megs (currently; on Chrome).
- You can only have one persistent database, in localStorage.
- Other databases will be cleared when the page is reloaded.
- You cannot use SQLite WASM and Cordova plugins at the same time: Use SQLite WASM for desktop apps and a Cordova plugin for mobile apps.
- You will have to export your old database and import it to the new one yourself. There is no way to do this automatically.
- The library adds about 1.4 megs to your app.
- SQLImport and SQLExport are not supported yet.
This workaround continues to evolve and is subject to change.